mirror of
https://github.com/szkolny-eu/szkolny-android.git
synced 2025-04-10 02:12:26 +02:00
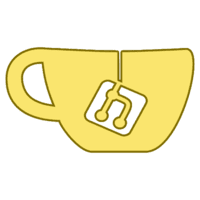
* [DB] Add Room schema export location. * [DB] Add Note entity and migration 96. * Add correct database schema * [Notes] Implement basic note list UI. * [DB] Implement Noteable in Full entities. Add note relation and filtering. * [Notes] Make Note searchable. * [UI] Disable onClick listeners in adapters when null. * [UI] Implement showing note list in dialog. * [UI] Update note dialogs UI. * [Notes] Add note details dialog. * [Notes] Extract note dialogs header into a separate layout. * [Notes] Add note editor dialog. * [Notes] Show note icons in dialogs and lists. * [Notes] Add showing substitute text. * [Notes] Add replacing notes icon. * [Notes] Add sharing and receiving notes. * [Notes] Add notes list UI fragment. * [Notes] Implement adding notes without owner. * [Notes] Add color names. * [Notes] Add notes card on home screen. * [Notes] Add notes card migration.
101 lines
2.7 KiB
Kotlin
101 lines
2.7 KiB
Kotlin
/*
|
|
* Copyright (c) Kuba Szczodrzyński 2021-10-17.
|
|
*/
|
|
|
|
package pl.szczodrzynski.edziennik.ext
|
|
|
|
import android.content.Context
|
|
import android.content.res.ColorStateList
|
|
import android.graphics.PorterDuff
|
|
import android.graphics.PorterDuffColorFilter
|
|
import android.graphics.drawable.Drawable
|
|
import android.util.TypedValue
|
|
import androidx.annotation.*
|
|
import androidx.core.content.res.ResourcesCompat
|
|
import com.mikepenz.iconics.typeface.IIcon
|
|
import pl.szczodrzynski.navlib.ImageHolder
|
|
|
|
fun colorFromName(name: String?): Int {
|
|
val i = (name ?: "").crc32()
|
|
return when ((i / 10 % 16 + 1).toInt()) {
|
|
13 -> 0xffF44336
|
|
4 -> 0xffF50057
|
|
2 -> 0xffD500F9
|
|
9 -> 0xff6200EA
|
|
5 -> 0xffFFAB00
|
|
1 -> 0xff304FFE
|
|
6 -> 0xff40C4FF
|
|
14 -> 0xff26A69A
|
|
15 -> 0xff00C853
|
|
7 -> 0xffFFD600
|
|
3 -> 0xffFF3D00
|
|
8 -> 0xffDD2C00
|
|
10 -> 0xff795548
|
|
12 -> 0xff2979FF
|
|
11 -> 0xffFF6D00
|
|
else -> 0xff64DD17
|
|
}.toInt()
|
|
}
|
|
|
|
fun colorFromCssName(name: String): Int {
|
|
return when (name) {
|
|
"red" -> 0xffff0000
|
|
"green" -> 0xff008000
|
|
"blue" -> 0xff0000ff
|
|
"violet" -> 0xffee82ee
|
|
"brown" -> 0xffa52a2a
|
|
"orange" -> 0xffffa500
|
|
"black" -> 0xff000000
|
|
"white" -> 0xffffffff
|
|
else -> -1L
|
|
}.toInt()
|
|
}
|
|
|
|
@ColorInt
|
|
fun @receiver:AttrRes Int.resolveAttr(context: Context?): Int {
|
|
val typedValue = TypedValue()
|
|
context?.theme?.resolveAttribute(this, typedValue, true)
|
|
return typedValue.data
|
|
}
|
|
@Dimension
|
|
fun @receiver:AttrRes Int.resolveDimenAttr(context: Context): Float {
|
|
val typedValue = TypedValue()
|
|
context.theme?.resolveAttribute(this, typedValue, true)
|
|
return typedValue.getDimension(context.resources.displayMetrics)
|
|
}
|
|
@ColorInt
|
|
fun @receiver:ColorRes Int.resolveColor(context: Context): Int {
|
|
return ResourcesCompat.getColor(context.resources, this, context.theme)
|
|
}
|
|
fun @receiver:DrawableRes Int.resolveDrawable(context: Context): Drawable {
|
|
return ResourcesCompat.getDrawable(context.resources, this, context.theme)!!
|
|
}
|
|
|
|
fun Int.toColorStateList(): ColorStateList {
|
|
val states = arrayOf(
|
|
intArrayOf( android.R.attr.state_enabled ),
|
|
intArrayOf(-android.R.attr.state_enabled ),
|
|
intArrayOf(-android.R.attr.state_checked ),
|
|
intArrayOf( android.R.attr.state_pressed )
|
|
)
|
|
|
|
val colors = intArrayOf(
|
|
this,
|
|
this,
|
|
this,
|
|
this
|
|
)
|
|
|
|
return ColorStateList(states, colors)
|
|
}
|
|
|
|
fun Drawable.setTintColor(color: Int): Drawable {
|
|
colorFilter = PorterDuffColorFilter(
|
|
color,
|
|
PorterDuff.Mode.SRC_ATOP
|
|
)
|
|
return this
|
|
}
|
|
|
|
fun IIcon.toImageHolder() = ImageHolder(this)
|