forked from github/wulkanowy-mirror
Add session expired dialog after password change (#389)
This commit is contained in:
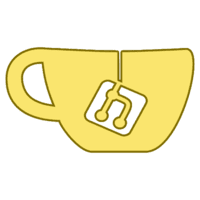
committed by
Mikołaj Pich
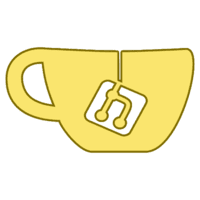
parent
0f75ff3206
commit
5c70cd8b8c
@ -1,8 +1,11 @@
|
||||
package io.github.wulkanowy.ui.base
|
||||
|
||||
import android.content.Intent.FLAG_ACTIVITY_CLEAR_TASK
|
||||
import android.content.Intent.FLAG_ACTIVITY_NEW_TASK
|
||||
import android.os.Bundle
|
||||
import android.view.View
|
||||
import android.widget.Toast
|
||||
import androidx.appcompat.app.AlertDialog
|
||||
import androidx.appcompat.app.AppCompatActivity
|
||||
import androidx.appcompat.app.AppCompatDelegate
|
||||
import androidx.fragment.app.Fragment
|
||||
@ -12,10 +15,12 @@ import dagger.android.AndroidInjection
|
||||
import dagger.android.DispatchingAndroidInjector
|
||||
import dagger.android.support.HasSupportFragmentInjector
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.ui.modules.login.LoginActivity
|
||||
import io.github.wulkanowy.utils.FragmentLifecycleLogger
|
||||
import javax.inject.Inject
|
||||
|
||||
abstract class BaseActivity : AppCompatActivity(), BaseView, HasSupportFragmentInjector {
|
||||
abstract class BaseActivity<T : BasePresenter<out BaseView>> : AppCompatActivity(), BaseView,
|
||||
HasSupportFragmentInjector {
|
||||
|
||||
@Inject
|
||||
lateinit var supportFragmentInjector: DispatchingAndroidInjector<Fragment>
|
||||
@ -28,6 +33,8 @@ abstract class BaseActivity : AppCompatActivity(), BaseView, HasSupportFragmentI
|
||||
|
||||
protected var messageContainer: View? = null
|
||||
|
||||
abstract var presenter: T
|
||||
|
||||
public override fun onCreate(savedInstanceState: Bundle?) {
|
||||
AndroidInjection.inject(this)
|
||||
themeManager.applyTheme(this)
|
||||
@ -51,9 +58,24 @@ abstract class BaseActivity : AppCompatActivity(), BaseView, HasSupportFragmentI
|
||||
else Toast.makeText(this, text, Toast.LENGTH_LONG).show()
|
||||
}
|
||||
|
||||
override fun showExpiredDialog() {
|
||||
AlertDialog.Builder(this)
|
||||
.setTitle(R.string.main_session_expired)
|
||||
.setMessage(R.string.main_session_relogin)
|
||||
.setPositiveButton(R.string.main_log_in) { _, _ -> presenter.onExpiredLoginSelected() }
|
||||
.setNegativeButton(android.R.string.cancel) { _, _ -> }
|
||||
.show()
|
||||
}
|
||||
|
||||
override fun openClearLoginView() {
|
||||
startActivity(LoginActivity.getStartIntent(this)
|
||||
.apply { addFlags(FLAG_ACTIVITY_CLEAR_TASK or FLAG_ACTIVITY_NEW_TASK) })
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
super.onDestroy()
|
||||
invalidateOptionsMenu()
|
||||
presenter.onDetachView()
|
||||
}
|
||||
|
||||
override fun supportFragmentInjector() = supportFragmentInjector
|
||||
|
@ -18,7 +18,7 @@ abstract class BaseFragment : DaggerFragment(), BaseView {
|
||||
}
|
||||
.show()
|
||||
} else {
|
||||
(activity as? BaseActivity)?.showError(text, error)
|
||||
(activity as? BaseActivity<*>)?.showError(text, error)
|
||||
}
|
||||
}
|
||||
|
||||
@ -26,7 +26,15 @@ abstract class BaseFragment : DaggerFragment(), BaseView {
|
||||
if (messageContainer != null) {
|
||||
Snackbar.make(messageContainer!!, text, LENGTH_LONG).show()
|
||||
} else {
|
||||
(activity as? BaseActivity)?.showMessage(text)
|
||||
(activity as? BaseActivity<*>)?.showMessage(text)
|
||||
}
|
||||
}
|
||||
|
||||
override fun showExpiredDialog() {
|
||||
(activity as? BaseActivity<*>)?.showExpiredDialog()
|
||||
}
|
||||
|
||||
override fun openClearLoginView() {
|
||||
(activity as? BaseActivity<*>)?.openClearLoginView()
|
||||
}
|
||||
}
|
||||
|
@ -1,8 +1,16 @@
|
||||
package io.github.wulkanowy.ui.base
|
||||
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.reactivex.Completable
|
||||
import io.reactivex.disposables.CompositeDisposable
|
||||
import timber.log.Timber
|
||||
|
||||
open class BasePresenter<T : BaseView>(private val errorHandler: ErrorHandler) {
|
||||
open class BasePresenter<T : BaseView>(
|
||||
protected val errorHandler: ErrorHandler,
|
||||
protected val studentRepository: StudentRepository,
|
||||
protected val schedulers: SchedulersProvider
|
||||
) {
|
||||
|
||||
val disposable = CompositeDisposable()
|
||||
|
||||
@ -10,7 +18,33 @@ open class BasePresenter<T : BaseView>(private val errorHandler: ErrorHandler) {
|
||||
|
||||
open fun onAttachView(view: T) {
|
||||
this.view = view
|
||||
errorHandler.showErrorMessage = { text, error -> view.showError(text, error) }
|
||||
errorHandler.apply {
|
||||
showErrorMessage = view::showError
|
||||
onSessionExpired = view::showExpiredDialog
|
||||
onNoCurrentStudent = view::openClearLoginView
|
||||
}
|
||||
}
|
||||
|
||||
fun onExpiredLoginSelected() {
|
||||
Timber.i("Attempt to switch the student after the session expires")
|
||||
disposable.add(studentRepository.getCurrentStudent(false)
|
||||
.flatMapCompletable { studentRepository.logoutStudent(it) }
|
||||
.andThen(studentRepository.getSavedStudents(false))
|
||||
.flatMapCompletable {
|
||||
if (it.isNotEmpty()) {
|
||||
Timber.i("Switching current student")
|
||||
studentRepository.switchStudent(it[0])
|
||||
} else Completable.complete()
|
||||
}
|
||||
.subscribeOn(schedulers.backgroundThread)
|
||||
.observeOn(schedulers.mainThread)
|
||||
.subscribe({
|
||||
Timber.i("Switch student result: Open login view")
|
||||
view?.openClearLoginView()
|
||||
}, {
|
||||
Timber.i("Switch student result: An exception occurred")
|
||||
errorHandler.dispatch(it)
|
||||
}))
|
||||
}
|
||||
|
||||
open fun onDetachView() {
|
||||
|
@ -5,4 +5,8 @@ interface BaseView {
|
||||
fun showError(text: String, error: Throwable)
|
||||
|
||||
fun showMessage(text: String)
|
||||
|
||||
fun showExpiredDialog()
|
||||
|
||||
fun openClearLoginView()
|
||||
}
|
||||
|
@ -5,7 +5,10 @@ import com.readystatesoftware.chuck.api.ChuckCollector
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.api.interceptor.FeatureDisabledException
|
||||
import io.github.wulkanowy.api.interceptor.ServiceUnavailableException
|
||||
import io.github.wulkanowy.api.login.BadCredentialsException
|
||||
import io.github.wulkanowy.api.login.NotLoggedInException
|
||||
import io.github.wulkanowy.data.exceptions.NoCurrentStudentException
|
||||
import io.github.wulkanowy.utils.security.ScramblerException
|
||||
import timber.log.Timber
|
||||
import java.net.SocketTimeoutException
|
||||
import java.net.UnknownHostException
|
||||
@ -15,6 +18,10 @@ open class ErrorHandler @Inject constructor(protected val resources: Resources,
|
||||
|
||||
var showErrorMessage: (String, Throwable) -> Unit = { _, _ -> }
|
||||
|
||||
var onSessionExpired: () -> Unit = {}
|
||||
|
||||
var onNoCurrentStudent: () -> Unit = {}
|
||||
|
||||
fun dispatch(error: Throwable) {
|
||||
chuckCollector.onError(error.javaClass.simpleName, error)
|
||||
Timber.e(error, "An exception occurred while the Wulkanowy was running")
|
||||
@ -22,17 +29,23 @@ open class ErrorHandler @Inject constructor(protected val resources: Resources,
|
||||
}
|
||||
|
||||
protected open fun proceed(error: Throwable) {
|
||||
showErrorMessage((when (error) {
|
||||
is UnknownHostException -> resources.getString(R.string.error_no_internet)
|
||||
is SocketTimeoutException -> resources.getString(R.string.error_timeout)
|
||||
is NotLoggedInException -> resources.getString(R.string.error_login_failed)
|
||||
is ServiceUnavailableException -> resources.getString(R.string.error_service_unavailable)
|
||||
is FeatureDisabledException -> resources.getString(R.string.error_feature_disabled)
|
||||
else -> resources.getString(R.string.error_unknown)
|
||||
}), error)
|
||||
resources.run {
|
||||
when (error) {
|
||||
is UnknownHostException -> showErrorMessage(getString(R.string.error_no_internet), error)
|
||||
is SocketTimeoutException -> showErrorMessage(getString(R.string.error_timeout), error)
|
||||
is NotLoggedInException -> showErrorMessage(getString(R.string.error_login_failed), error)
|
||||
is ServiceUnavailableException -> showErrorMessage(getString(R.string.error_service_unavailable), error)
|
||||
is FeatureDisabledException -> showErrorMessage(getString(R.string.error_feature_disabled), error)
|
||||
is ScramblerException, is BadCredentialsException -> onSessionExpired()
|
||||
is NoCurrentStudentException -> onNoCurrentStudent()
|
||||
else -> showErrorMessage(getString(R.string.error_unknown), error)
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
open fun clear() {
|
||||
showErrorMessage = { _, _ -> }
|
||||
onSessionExpired = {}
|
||||
onNoCurrentStudent = {}
|
||||
}
|
||||
}
|
||||
|
@ -1,21 +0,0 @@
|
||||
package io.github.wulkanowy.ui.base.session
|
||||
|
||||
import android.content.Intent.FLAG_ACTIVITY_CLEAR_TASK
|
||||
import android.content.Intent.FLAG_ACTIVITY_NEW_TASK
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.login.LoginActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
|
||||
open class BaseSessionFragment : BaseFragment(), BaseSessionView {
|
||||
|
||||
override fun showExpiredDialog() {
|
||||
(activity as? MainActivity)?.showExpiredDialog()
|
||||
}
|
||||
|
||||
override fun openLoginView() {
|
||||
activity?.also {
|
||||
startActivity(LoginActivity.getStartIntent(it)
|
||||
.apply { addFlags(FLAG_ACTIVITY_CLEAR_TASK or FLAG_ACTIVITY_NEW_TASK) })
|
||||
}
|
||||
}
|
||||
}
|
@ -1,15 +0,0 @@
|
||||
package io.github.wulkanowy.ui.base.session
|
||||
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
|
||||
open class BaseSessionPresenter<T : BaseSessionView>(private val errorHandler: SessionErrorHandler) :
|
||||
BasePresenter<T>(errorHandler) {
|
||||
|
||||
override fun onAttachView(view: T) {
|
||||
super.onAttachView(view)
|
||||
errorHandler.apply {
|
||||
onDecryptionFail = { view.showExpiredDialog() }
|
||||
onNoCurrentStudent = { view.openLoginView() }
|
||||
}
|
||||
}
|
||||
}
|
@ -1,10 +0,0 @@
|
||||
package io.github.wulkanowy.ui.base.session
|
||||
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface BaseSessionView : BaseView {
|
||||
|
||||
fun showExpiredDialog()
|
||||
|
||||
fun openLoginView()
|
||||
}
|
@ -1,32 +0,0 @@
|
||||
package io.github.wulkanowy.ui.base.session
|
||||
|
||||
import android.content.res.Resources
|
||||
import com.readystatesoftware.chuck.api.ChuckCollector
|
||||
import io.github.wulkanowy.data.exceptions.NoCurrentStudentException
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.security.ScramblerException
|
||||
import javax.inject.Inject
|
||||
|
||||
open class SessionErrorHandler @Inject constructor(
|
||||
resources: Resources,
|
||||
chuckCollector: ChuckCollector
|
||||
) : ErrorHandler(resources, chuckCollector) {
|
||||
|
||||
var onDecryptionFail: () -> Unit = {}
|
||||
|
||||
var onNoCurrentStudent: () -> Unit = {}
|
||||
|
||||
override fun proceed(error: Throwable) {
|
||||
when (error) {
|
||||
is ScramblerException -> onDecryptionFail()
|
||||
is NoCurrentStudentException -> onNoCurrentStudent()
|
||||
else -> super.proceed(error)
|
||||
}
|
||||
}
|
||||
|
||||
override fun clear() {
|
||||
super.clear()
|
||||
onDecryptionFail = {}
|
||||
onNoCurrentStudent = {}
|
||||
}
|
||||
}
|
@ -4,16 +4,20 @@ import com.mikepenz.aboutlibraries.Libs
|
||||
import com.mikepenz.aboutlibraries.Libs.SpecialButton.SPECIAL1
|
||||
import com.mikepenz.aboutlibraries.Libs.SpecialButton.SPECIAL2
|
||||
import com.mikepenz.aboutlibraries.Libs.SpecialButton.SPECIAL3
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class AboutPresenter @Inject constructor(
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<AboutView>(errorHandler) {
|
||||
) : BasePresenter<AboutView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: AboutView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -1,7 +1,5 @@
|
||||
package io.github.wulkanowy.ui.modules.account
|
||||
|
||||
import android.content.Intent.FLAG_ACTIVITY_CLEAR_TASK
|
||||
import android.content.Intent.FLAG_ACTIVITY_NEW_TASK
|
||||
import android.os.Bundle
|
||||
import android.view.LayoutInflater
|
||||
import android.view.View
|
||||
@ -14,6 +12,7 @@ import eu.davidea.flexibleadapter.FlexibleAdapter
|
||||
import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.ui.base.BaseActivity
|
||||
import io.github.wulkanowy.ui.modules.login.LoginActivity
|
||||
import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.dialog_account.*
|
||||
@ -73,16 +72,17 @@ class AccountDialog : DaggerAppCompatDialogFragment(), AccountView {
|
||||
}
|
||||
|
||||
override fun openLoginView() {
|
||||
activity?.also {
|
||||
activity?.let {
|
||||
startActivity(LoginActivity.getStartIntent(it))
|
||||
}
|
||||
}
|
||||
|
||||
override fun showExpiredDialog() {
|
||||
(activity as? BaseActivity<*>)?.showExpiredDialog()
|
||||
}
|
||||
|
||||
override fun openClearLoginView() {
|
||||
activity?.also {
|
||||
startActivity(LoginActivity.getStartIntent(it)
|
||||
.apply { addFlags(FLAG_ACTIVITY_CLEAR_TASK or FLAG_ACTIVITY_NEW_TASK) })
|
||||
}
|
||||
(activity as? BaseActivity<*>)?.openClearLoginView()
|
||||
}
|
||||
|
||||
override fun showConfirmDialog() {
|
||||
|
@ -11,11 +11,11 @@ import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class AccountPresenter @Inject constructor(
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val syncManager: SyncManager,
|
||||
private val schedulers: SchedulersProvider
|
||||
) : BasePresenter<AccountView>(errorHandler) {
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val syncManager: SyncManager
|
||||
) : BasePresenter<AccountView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: AccountView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -14,8 +14,6 @@ interface AccountView : BaseView {
|
||||
|
||||
fun openLoginView()
|
||||
|
||||
fun openClearLoginView()
|
||||
|
||||
fun recreateMainView()
|
||||
}
|
||||
|
||||
|
@ -13,7 +13,7 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Attendance
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.attendance.summary.AttendanceSummaryFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
@ -21,7 +21,7 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_attendance.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class AttendanceFragment : BaseSessionFragment(), AttendanceView, MainView.MainChildView, MainView.TitledView {
|
||||
class AttendanceFragment : BaseFragment(), AttendanceView, MainView.MainChildView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: AttendancePresenter
|
||||
|
@ -6,8 +6,8 @@ import io.github.wulkanowy.data.repositories.attendance.AttendanceRepository
|
||||
import io.github.wulkanowy.data.repositories.preferences.PreferencesRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.isHolidays
|
||||
@ -23,14 +23,14 @@ import java.util.concurrent.TimeUnit.MILLISECONDS
|
||||
import javax.inject.Inject
|
||||
|
||||
class AttendancePresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val attendanceRepository: AttendanceRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val prefRepository: PreferencesRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<AttendanceView>(errorHandler) {
|
||||
) : BasePresenter<AttendanceView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var currentDate: LocalDate
|
||||
private set
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.attendance
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Attendance
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface AttendanceView : BaseSessionView {
|
||||
interface AttendanceView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -13,13 +13,13 @@ import eu.davidea.flexibleadapter.FlexibleAdapter
|
||||
import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.utils.setOnItemSelectedListener
|
||||
import kotlinx.android.synthetic.main.fragment_attendance_summary.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class AttendanceSummaryFragment : BaseSessionFragment(), AttendanceSummaryView, MainView.TitledView {
|
||||
class AttendanceSummaryFragment : BaseFragment(), AttendanceSummaryView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: AttendanceSummaryPresenter
|
||||
|
@ -6,8 +6,8 @@ import io.github.wulkanowy.data.repositories.attendancesummary.AttendanceSummary
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.data.repositories.subject.SubjectRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.calculatePercentage
|
||||
@ -19,14 +19,14 @@ import java.util.concurrent.TimeUnit.MILLISECONDS
|
||||
import javax.inject.Inject
|
||||
|
||||
class AttendanceSummaryPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val attendanceSummaryRepository: AttendanceSummaryRepository,
|
||||
private val subjectRepository: SubjectRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<AttendanceSummaryView>(errorHandler) {
|
||||
) : BasePresenter<AttendanceSummaryView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
private var subjects = emptyList<Subject>()
|
||||
|
||||
|
@ -1,8 +1,8 @@
|
||||
package io.github.wulkanowy.ui.modules.attendance.summary
|
||||
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface AttendanceSummaryView : BaseSessionView {
|
||||
interface AttendanceSummaryView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -13,14 +13,14 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Exam
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_exam.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class ExamFragment : BaseSessionFragment(), ExamView, MainView.MainChildView, MainView.TitledView {
|
||||
class ExamFragment : BaseFragment(), ExamView, MainView.MainChildView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: ExamPresenter
|
||||
|
@ -6,8 +6,8 @@ import io.github.wulkanowy.data.db.entities.Exam
|
||||
import io.github.wulkanowy.data.repositories.exam.ExamRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.friday
|
||||
@ -23,13 +23,13 @@ import java.util.concurrent.TimeUnit.MILLISECONDS
|
||||
import javax.inject.Inject
|
||||
|
||||
class ExamPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val examRepository: ExamRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<ExamView>(errorHandler) {
|
||||
) : BasePresenter<ExamView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var currentDate: LocalDate
|
||||
private set
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.exam
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Exam
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface ExamView : BaseSessionView {
|
||||
interface ExamView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -11,8 +11,8 @@ import android.view.View.VISIBLE
|
||||
import android.view.ViewGroup
|
||||
import androidx.appcompat.app.AlertDialog
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragmentPagerAdapter
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.details.GradeDetailsFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.statistics.GradeStatisticsFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.summary.GradeSummaryFragment
|
||||
@ -21,7 +21,7 @@ import io.github.wulkanowy.utils.setOnSelectPageListener
|
||||
import kotlinx.android.synthetic.main.fragment_grade.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeFragment : BaseSessionFragment(), GradeView, MainView.MainChildView, MainView.TitledView {
|
||||
class GradeFragment : BaseFragment(), GradeView, MainView.MainChildView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: GradePresenter
|
||||
|
@ -3,20 +3,20 @@ package io.github.wulkanowy.ui.modules.grade
|
||||
import io.github.wulkanowy.data.db.entities.Semester
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradePresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<GradeView>(errorHandler) {
|
||||
) : BasePresenter<GradeView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
var selectedIndex = 0
|
||||
private set
|
||||
|
@ -1,8 +1,8 @@
|
||||
package io.github.wulkanowy.ui.modules.grade
|
||||
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface GradeView : BaseSessionView {
|
||||
interface GradeView : BaseView {
|
||||
|
||||
val currentPageIndex: Int
|
||||
|
||||
|
@ -17,7 +17,7 @@ import eu.davidea.flexibleadapter.items.IExpandable
|
||||
import eu.davidea.flexibleadapter.items.IFlexible
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Grade
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeView
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
@ -25,7 +25,7 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_grade_details.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeDetailsFragment : BaseSessionFragment(), GradeDetailsView, GradeView.GradeChildView {
|
||||
class GradeDetailsFragment : BaseFragment(), GradeDetailsView, GradeView.GradeChildView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: GradeDetailsPresenter
|
||||
|
@ -6,8 +6,8 @@ import io.github.wulkanowy.data.repositories.grade.GradeRepository
|
||||
import io.github.wulkanowy.data.repositories.preferences.PreferencesRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeAverageProvider
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
@ -16,15 +16,15 @@ import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeDetailsPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val gradeRepository: GradeRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val preferencesRepository: PreferencesRepository,
|
||||
private val averageProvider: GradeAverageProvider,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<GradeDetailsView>(errorHandler) {
|
||||
) : BasePresenter<GradeDetailsView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
private var currentSemesterId = 0
|
||||
|
||||
|
@ -4,9 +4,9 @@ import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import eu.davidea.flexibleadapter.items.IExpandable
|
||||
import eu.davidea.flexibleadapter.items.IFlexible
|
||||
import io.github.wulkanowy.data.db.entities.Grade
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface GradeDetailsView : BaseSessionView {
|
||||
interface GradeDetailsView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -16,7 +16,7 @@ import com.github.mikephil.charting.data.PieEntry
|
||||
import com.github.mikephil.charting.formatter.ValueFormatter
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.GradeStatistics
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeView
|
||||
import io.github.wulkanowy.utils.getThemeAttrColor
|
||||
@ -24,7 +24,7 @@ import io.github.wulkanowy.utils.setOnItemSelectedListener
|
||||
import kotlinx.android.synthetic.main.fragment_grade_statistics.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeStatisticsFragment : BaseSessionFragment(), GradeStatisticsView, GradeView.GradeChildView {
|
||||
class GradeStatisticsFragment : BaseFragment(), GradeStatisticsView, GradeView.GradeChildView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: GradeStatisticsPresenter
|
||||
|
@ -6,23 +6,23 @@ import io.github.wulkanowy.data.repositories.preferences.PreferencesRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.data.repositories.subject.SubjectRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeStatisticsPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val gradeStatisticsRepository: GradeStatisticsRepository,
|
||||
private val subjectRepository: SubjectRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val preferencesRepository: PreferencesRepository,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<GradeStatisticsView>(errorHandler) {
|
||||
) : BasePresenter<GradeStatisticsView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
private var subjects = emptyList<Subject>()
|
||||
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.grade.statistics
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.GradeStatistics
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface GradeStatisticsView : BaseSessionView {
|
||||
interface GradeStatisticsView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -11,13 +11,13 @@ import eu.davidea.flexibleadapter.FlexibleAdapter
|
||||
import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeView
|
||||
import kotlinx.android.synthetic.main.fragment_grade_summary.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeSummaryFragment : BaseSessionFragment(), GradeSummaryView, GradeView.GradeChildView {
|
||||
class GradeSummaryFragment : BaseFragment(), GradeSummaryView, GradeView.GradeChildView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: GradeSummaryPresenter
|
||||
|
@ -4,8 +4,8 @@ import io.github.wulkanowy.data.db.entities.GradeSummary
|
||||
import io.github.wulkanowy.data.repositories.gradessummary.GradeSummaryRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeAverageProvider
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
@ -16,14 +16,14 @@ import java.util.Locale.FRANCE
|
||||
import javax.inject.Inject
|
||||
|
||||
class GradeSummaryPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val gradeSummaryRepository: GradeSummaryRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val averageProvider: GradeAverageProvider,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<GradeSummaryView>(errorHandler) {
|
||||
) : BasePresenter<GradeSummaryView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: GradeSummaryView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -1,8 +1,8 @@
|
||||
package io.github.wulkanowy.ui.modules.grade.summary
|
||||
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface GradeSummaryView : BaseSessionView {
|
||||
interface GradeSummaryView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -10,14 +10,14 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Homework
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_homework.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class HomeworkFragment : BaseSessionFragment(), HomeworkView, MainView.TitledView {
|
||||
class HomeworkFragment : BaseFragment(), HomeworkView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: HomeworkPresenter
|
||||
|
@ -6,8 +6,8 @@ import io.github.wulkanowy.data.db.entities.Homework
|
||||
import io.github.wulkanowy.data.repositories.homework.HomeworkRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.friday
|
||||
@ -21,13 +21,13 @@ import java.util.concurrent.TimeUnit
|
||||
import javax.inject.Inject
|
||||
|
||||
class HomeworkPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val homeworkRepository: HomeworkRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<HomeworkView>(errorHandler) {
|
||||
) : BasePresenter<HomeworkView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var currentDate: LocalDate
|
||||
private set
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.homework
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Homework
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface HomeworkView : BaseSessionView {
|
||||
interface HomeworkView : BaseView {
|
||||
|
||||
fun initView()
|
||||
|
||||
|
@ -14,10 +14,10 @@ import io.github.wulkanowy.utils.setOnSelectPageListener
|
||||
import kotlinx.android.synthetic.main.activity_login.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class LoginActivity : BaseActivity(), LoginView {
|
||||
class LoginActivity : BaseActivity<LoginPresenter>(), LoginView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: LoginPresenter
|
||||
override lateinit var presenter: LoginPresenter
|
||||
|
||||
@Inject
|
||||
lateinit var loginAdapter: BaseFragmentPagerAdapter
|
||||
@ -81,9 +81,4 @@ class LoginActivity : BaseActivity(), LoginView {
|
||||
fun onSymbolFragmentAccountLogged(students: List<Student>) {
|
||||
presenter.onSymbolViewAccountLogged(students)
|
||||
}
|
||||
|
||||
public override fun onDestroy() {
|
||||
presenter.onDetachView()
|
||||
super.onDestroy()
|
||||
}
|
||||
}
|
||||
|
@ -1,12 +1,18 @@
|
||||
package io.github.wulkanowy.ui.modules.login
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Student
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class LoginPresenter @Inject constructor(errorHandler: ErrorHandler) : BasePresenter<LoginView>(errorHandler) {
|
||||
class LoginPresenter @Inject constructor(
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository
|
||||
) : BasePresenter<LoginView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: LoginView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -11,12 +11,12 @@ import javax.inject.Inject
|
||||
import javax.inject.Named
|
||||
|
||||
class LoginFormPresenter @Inject constructor(
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val errorHandler: LoginErrorHandler,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
studentRepository: StudentRepository,
|
||||
private val loginErrorHandler: LoginErrorHandler,
|
||||
private val analytics: FirebaseAnalyticsHelper,
|
||||
@param:Named("isDebug") private val isDebug: Boolean
|
||||
) : BasePresenter<LoginFormView>(errorHandler) {
|
||||
) : BasePresenter<LoginFormView>(loginErrorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: LoginFormView) {
|
||||
super.onAttachView(view)
|
||||
@ -24,7 +24,7 @@ class LoginFormPresenter @Inject constructor(
|
||||
initView()
|
||||
if (isDebug) showVersion() else showPrivacyPolicy()
|
||||
|
||||
errorHandler.onBadCredentials = {
|
||||
loginErrorHandler.onBadCredentials = {
|
||||
setErrorPassIncorrect()
|
||||
showSoftKeyboard()
|
||||
Timber.i("Entered wrong username or password")
|
||||
@ -83,7 +83,7 @@ class LoginFormPresenter @Inject constructor(
|
||||
}, {
|
||||
Timber.i("Login result: An exception occurred")
|
||||
analytics.logEvent("registration_form", SUCCESS to false, "students" to -1, "endpoint" to endpoint, "error" to it.localizedMessage)
|
||||
errorHandler.dispatch(it)
|
||||
loginErrorHandler.dispatch(it)
|
||||
}))
|
||||
}
|
||||
|
||||
|
@ -13,11 +13,11 @@ import java.io.Serializable
|
||||
import javax.inject.Inject
|
||||
|
||||
class LoginStudentSelectPresenter @Inject constructor(
|
||||
private val errorHandler: LoginErrorHandler,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
studentRepository: StudentRepository,
|
||||
private val loginErrorHandler: LoginErrorHandler,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<LoginStudentSelectView>(errorHandler) {
|
||||
) : BasePresenter<LoginStudentSelectView>(loginErrorHandler, studentRepository, schedulers) {
|
||||
|
||||
var students = emptyList<Student>()
|
||||
|
||||
@ -28,7 +28,7 @@ class LoginStudentSelectPresenter @Inject constructor(
|
||||
view.run {
|
||||
initView()
|
||||
enableSignIn(false)
|
||||
errorHandler.onStudentDuplicate = {
|
||||
loginErrorHandler.onStudentDuplicate = {
|
||||
showMessage(it)
|
||||
Timber.i("The student already registered in the app was selected")
|
||||
}
|
||||
@ -84,7 +84,7 @@ class LoginStudentSelectPresenter @Inject constructor(
|
||||
}, { error ->
|
||||
students.forEach { analytics.logEvent("registration_student_select", SUCCESS to false, "endpoint" to it.endpoint, "symbol" to it.symbol, "error" to error.localizedMessage) }
|
||||
Timber.i("Registration result: An exception occurred ")
|
||||
errorHandler.dispatch(error)
|
||||
loginErrorHandler.dispatch(error)
|
||||
view?.apply {
|
||||
showProgress(false)
|
||||
showContent(true)
|
||||
|
@ -12,11 +12,11 @@ import java.io.Serializable
|
||||
import javax.inject.Inject
|
||||
|
||||
class LoginSymbolPresenter @Inject constructor(
|
||||
private val studentRepository: StudentRepository,
|
||||
private val errorHandler: LoginErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
private val loginErrorHandler: LoginErrorHandler,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<LoginSymbolView>(errorHandler) {
|
||||
) : BasePresenter<LoginSymbolView>(loginErrorHandler, studentRepository, schedulers) {
|
||||
|
||||
var loginData: Triple<String, String, String>? = null
|
||||
|
||||
@ -72,7 +72,7 @@ class LoginSymbolPresenter @Inject constructor(
|
||||
}, {
|
||||
Timber.i("Login with symbol result: An exception occurred")
|
||||
analytics.logEvent("registration_symbol", SUCCESS to false, "students" to -1, "endpoint" to loginData?.third, "symbol" to symbol, "error" to it.localizedMessage)
|
||||
errorHandler.dispatch(it)
|
||||
loginErrorHandler.dispatch(it)
|
||||
}))
|
||||
}
|
||||
|
||||
|
@ -6,12 +6,12 @@ import android.view.View
|
||||
import android.view.ViewGroup
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.LuckyNumber
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import kotlinx.android.synthetic.main.fragment_lucky_number.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class LuckyNumberFragment : BaseSessionFragment(), LuckyNumberView, MainView.TitledView {
|
||||
class LuckyNumberFragment : BaseFragment(), LuckyNumberView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: LuckyNumberPresenter
|
||||
|
@ -4,20 +4,20 @@ import io.github.wulkanowy.data.repositories.luckynumber.LuckyNumberRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class LuckyNumberPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val luckyNumberRepository: LuckyNumberRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<LuckyNumberView>(errorHandler) {
|
||||
) : BasePresenter<LuckyNumberView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: LuckyNumberView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.luckynumber
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.LuckyNumber
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface LuckyNumberView : BaseSessionView {
|
||||
interface LuckyNumberView : BaseView {
|
||||
|
||||
fun initView()
|
||||
|
||||
|
@ -16,13 +16,14 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.activity_widget_configure.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class LuckyNumberWidgetConfigureActivity : BaseActivity(), LuckyNumberWidgetConfigureView {
|
||||
class LuckyNumberWidgetConfigureActivity : BaseActivity<LuckyNumberWidgetConfigurePresenter>(),
|
||||
LuckyNumberWidgetConfigureView {
|
||||
|
||||
@Inject
|
||||
lateinit var configureAdapter: FlexibleAdapter<AbstractFlexibleItem<*>>
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: LuckyNumberWidgetConfigurePresenter
|
||||
override lateinit var presenter: LuckyNumberWidgetConfigurePresenter
|
||||
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
@ -69,9 +70,4 @@ class LuckyNumberWidgetConfigureActivity : BaseActivity(), LuckyNumberWidgetConf
|
||||
override fun openLoginView() {
|
||||
startActivity(LoginActivity.getStartIntent(this))
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
super.onDestroy()
|
||||
presenter.onDetachView()
|
||||
}
|
||||
}
|
||||
|
@ -11,11 +11,11 @@ import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import javax.inject.Inject
|
||||
|
||||
class LuckyNumberWidgetConfigurePresenter @Inject constructor(
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val sharedPref: SharedPrefHelper
|
||||
) : BasePresenter<LuckyNumberWidgetConfigureView>(errorHandler) {
|
||||
) : BasePresenter<LuckyNumberWidgetConfigureView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
private var appWidgetId: Int? = null
|
||||
|
||||
|
@ -7,7 +7,6 @@ import android.content.Intent.FLAG_ACTIVITY_NEW_TASK
|
||||
import android.os.Bundle
|
||||
import android.view.Menu
|
||||
import android.view.MenuItem
|
||||
import androidx.appcompat.app.AlertDialog
|
||||
import androidx.core.content.ContextCompat
|
||||
import androidx.fragment.app.DialogFragment
|
||||
import androidx.fragment.app.Fragment
|
||||
@ -22,7 +21,6 @@ import io.github.wulkanowy.ui.modules.attendance.AttendanceFragment
|
||||
import io.github.wulkanowy.ui.modules.exam.ExamFragment
|
||||
import io.github.wulkanowy.ui.modules.grade.GradeFragment
|
||||
import io.github.wulkanowy.ui.modules.homework.HomeworkFragment
|
||||
import io.github.wulkanowy.ui.modules.login.LoginActivity
|
||||
import io.github.wulkanowy.ui.modules.luckynumber.LuckyNumberFragment
|
||||
import io.github.wulkanowy.ui.modules.message.MessageFragment
|
||||
import io.github.wulkanowy.ui.modules.more.MoreFragment
|
||||
@ -34,10 +32,10 @@ import io.github.wulkanowy.utils.setOnViewChangeListener
|
||||
import kotlinx.android.synthetic.main.activity_main.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class MainActivity : BaseActivity(), MainView {
|
||||
class MainActivity : BaseActivity<MainPresenter>(), MainView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: MainPresenter
|
||||
override lateinit var presenter: MainPresenter
|
||||
|
||||
@Inject
|
||||
lateinit var navController: FragNavController
|
||||
@ -154,15 +152,6 @@ class MainActivity : BaseActivity(), MainView {
|
||||
navController.showDialogFragment(AccountDialog.newInstance())
|
||||
}
|
||||
|
||||
fun showExpiredDialog() {
|
||||
AlertDialog.Builder(this)
|
||||
.setTitle(R.string.main_session_expired)
|
||||
.setMessage(R.string.main_session_relogin)
|
||||
.setPositiveButton(R.string.main_log_in) { _, _ -> presenter.onLoginSelected() }
|
||||
.setNegativeButton(android.R.string.cancel) { _, _ -> }
|
||||
.show()
|
||||
}
|
||||
|
||||
override fun notifyMenuViewReselected() {
|
||||
(navController.currentStack?.getOrNull(0) as? MainView.MainChildView)?.onFragmentReselected()
|
||||
}
|
||||
@ -183,19 +172,9 @@ class MainActivity : BaseActivity(), MainView {
|
||||
presenter.onBackPressed { super.onBackPressed() }
|
||||
}
|
||||
|
||||
override fun openLoginView() {
|
||||
startActivity(LoginActivity.getStartIntent(this)
|
||||
.apply { addFlags(FLAG_ACTIVITY_CLEAR_TASK or FLAG_ACTIVITY_NEW_TASK) })
|
||||
}
|
||||
|
||||
override fun onSaveInstanceState(outState: Bundle) {
|
||||
super.onSaveInstanceState(outState)
|
||||
navController.onSaveInstanceState(outState)
|
||||
intent.removeExtra(EXTRA_START_MENU)
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
super.onDestroy()
|
||||
presenter.onDetachView()
|
||||
}
|
||||
}
|
||||
|
@ -9,18 +9,17 @@ import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.reactivex.Completable
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class MainPresenter @Inject constructor(
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val prefRepository: PreferencesRepository,
|
||||
private val syncManager: SyncManager,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<MainView>(errorHandler) {
|
||||
) : BasePresenter<MainView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
fun onAttachView(view: MainView, initMenu: MainView.MenuView?) {
|
||||
super.onAttachView(view)
|
||||
@ -80,28 +79,6 @@ class MainPresenter @Inject constructor(
|
||||
} == true
|
||||
}
|
||||
|
||||
fun onLoginSelected() {
|
||||
Timber.i("Attempt to switch the student after the session expires")
|
||||
disposable.add(studentRepository.getCurrentStudent(false)
|
||||
.flatMapCompletable { studentRepository.logoutStudent(it) }
|
||||
.andThen(studentRepository.getSavedStudents(false))
|
||||
.flatMapCompletable {
|
||||
if (it.isNotEmpty()) {
|
||||
Timber.i("Switching current student")
|
||||
studentRepository.switchStudent(it[0])
|
||||
} else Completable.complete()
|
||||
}
|
||||
.subscribeOn(schedulers.backgroundThread)
|
||||
.observeOn(schedulers.mainThread)
|
||||
.subscribe({
|
||||
Timber.i("Switch student result: Open login view")
|
||||
view?.openLoginView()
|
||||
}, {
|
||||
Timber.i("Switch student result: An exception occurred")
|
||||
errorHandler.dispatch(it)
|
||||
}))
|
||||
}
|
||||
|
||||
private fun getProperViewIndexes(initMenu: MainView.MenuView?): Pair<Int, Int> {
|
||||
return when {
|
||||
initMenu?.id in 0..3 -> initMenu!!.id to -1
|
||||
|
@ -28,8 +28,6 @@ interface MainView : BaseView {
|
||||
|
||||
fun popView()
|
||||
|
||||
fun openLoginView()
|
||||
|
||||
interface MainChildView {
|
||||
|
||||
fun onFragmentReselected()
|
||||
|
@ -1,6 +1,7 @@
|
||||
package io.github.wulkanowy.ui.modules.message
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Message
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
@ -10,9 +11,10 @@ import java.util.concurrent.TimeUnit.MILLISECONDS
|
||||
import javax.inject.Inject
|
||||
|
||||
class MessagePresenter @Inject constructor(
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
private val schedulers: SchedulersProvider
|
||||
) : BasePresenter<MessageView>(errorHandler) {
|
||||
studentRepository: StudentRepository
|
||||
) : BasePresenter<MessageView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: MessageView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -12,7 +12,7 @@ import android.view.View.VISIBLE
|
||||
import android.view.ViewGroup
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Message
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.ui.modules.message.MessageFragment
|
||||
@ -20,8 +20,7 @@ import io.github.wulkanowy.ui.modules.message.send.SendMessageActivity
|
||||
import kotlinx.android.synthetic.main.fragment_message_preview.*
|
||||
import javax.inject.Inject
|
||||
|
||||
@SuppressLint("SetTextI18n")
|
||||
class MessagePreviewFragment : BaseSessionFragment(), MessagePreviewView, MainView.TitledView {
|
||||
class MessagePreviewFragment : BaseFragment(), MessagePreviewView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: MessagePreviewPresenter
|
||||
@ -87,10 +86,12 @@ class MessagePreviewFragment : BaseSessionFragment(), MessagePreviewView, MainVi
|
||||
messagePreviewSubject.text = subject
|
||||
}
|
||||
|
||||
@SuppressLint("SetTextI18n")
|
||||
override fun setRecipient(recipient: String) {
|
||||
messagePreviewAuthor.text = "${getString(R.string.message_to)} $recipient"
|
||||
}
|
||||
|
||||
@SuppressLint("SetTextI18n")
|
||||
override fun setSender(sender: String) {
|
||||
messagePreviewAuthor.text = "${getString(R.string.message_from)} $sender"
|
||||
}
|
||||
|
@ -4,8 +4,8 @@ import com.google.firebase.analytics.FirebaseAnalytics.Param.START_DATE
|
||||
import io.github.wulkanowy.data.db.entities.Message
|
||||
import io.github.wulkanowy.data.repositories.message.MessageRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.toFormattedString
|
||||
@ -13,12 +13,12 @@ import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class MessagePreviewPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val messageRepository: MessageRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<MessagePreviewView>(errorHandler) {
|
||||
) : BasePresenter<MessagePreviewView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
var messageId = 0L
|
||||
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.message.preview
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Message
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface MessagePreviewView : BaseSessionView {
|
||||
interface MessagePreviewView : BaseView {
|
||||
|
||||
val noSubjectString: String
|
||||
|
||||
|
@ -19,13 +19,14 @@ import io.github.wulkanowy.utils.showSoftInput
|
||||
import kotlinx.android.synthetic.main.activity_send_message.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class SendMessageActivity : BaseActivity(), SendMessageView {
|
||||
class SendMessageActivity : BaseActivity<SendMessagePresenter>(), SendMessageView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: SendMessagePresenter
|
||||
override lateinit var presenter: SendMessagePresenter
|
||||
|
||||
companion object {
|
||||
private const val EXTRA_MESSAGE = "EXTRA_MESSAGE"
|
||||
|
||||
private const val EXTRA_REPLY = "EXTRA_REPLY"
|
||||
|
||||
fun getStartIntent(context: Context) = Intent(context, SendMessageActivity::class.java)
|
||||
@ -126,9 +127,4 @@ class SendMessageActivity : BaseActivity(), SendMessageView {
|
||||
override fun popView() {
|
||||
onBackPressed()
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
presenter.onDetachView()
|
||||
super.onDestroy()
|
||||
}
|
||||
}
|
||||
|
@ -19,16 +19,16 @@ import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class SendMessagePresenter @Inject constructor(
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val messageRepository: MessageRepository,
|
||||
private val reportingUnitRepository: ReportingUnitRepository,
|
||||
private val recipientRepository: RecipientRepository,
|
||||
private val preferencesRepository: PreferencesRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BasePresenter<SendMessageView>(errorHandler) {
|
||||
) : BasePresenter<SendMessageView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
fun onAttachView(view: SendMessageView, message: Message?, reply: Boolean?) {
|
||||
super.onAttachView(view)
|
||||
|
@ -13,7 +13,7 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.repositories.message.MessageFolder
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.message.MessageFragment
|
||||
import io.github.wulkanowy.ui.modules.message.MessageItem
|
||||
@ -22,7 +22,7 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_message_tab.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class MessageTabFragment : BaseSessionFragment(), MessageTabView {
|
||||
class MessageTabFragment : BaseFragment(), MessageTabView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: MessageTabPresenter
|
||||
|
@ -5,8 +5,8 @@ import io.github.wulkanowy.data.db.entities.Message
|
||||
import io.github.wulkanowy.data.repositories.message.MessageFolder
|
||||
import io.github.wulkanowy.data.repositories.message.MessageRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.ui.modules.message.MessageItem
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
@ -14,12 +14,12 @@ import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class MessageTabPresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val messageRepository: MessageRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<MessageTabView>(errorHandler) {
|
||||
) : BasePresenter<MessageTabView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var folder: MessageFolder
|
||||
|
||||
|
@ -1,10 +1,10 @@
|
||||
package io.github.wulkanowy.ui.modules.message.tab
|
||||
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
import io.github.wulkanowy.ui.modules.message.MessageItem
|
||||
|
||||
interface MessageTabView : BaseSessionView {
|
||||
interface MessageTabView : BaseView {
|
||||
|
||||
val noSubjectString: String
|
||||
|
||||
|
@ -1,12 +1,18 @@
|
||||
package io.github.wulkanowy.ui.modules.more
|
||||
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class MorePresenter @Inject constructor(errorHandler: ErrorHandler) : BasePresenter<MoreView>(errorHandler) {
|
||||
class MorePresenter @Inject constructor(
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository
|
||||
) : BasePresenter<MoreView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: MoreView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -12,14 +12,14 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Note
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_note.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class NoteFragment : BaseSessionFragment(), NoteView, MainView.TitledView {
|
||||
class NoteFragment : BaseFragment(), NoteView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: NotePresenter
|
||||
|
@ -5,21 +5,21 @@ import io.github.wulkanowy.data.db.entities.Note
|
||||
import io.github.wulkanowy.data.repositories.note.NoteRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class NotePresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val noteRepository: NoteRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<NoteView>(errorHandler) {
|
||||
) : BasePresenter<NoteView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: NoteView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -2,9 +2,9 @@ package io.github.wulkanowy.ui.modules.note
|
||||
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.data.db.entities.Note
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface NoteView : BaseSessionView {
|
||||
interface NoteView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -55,11 +55,19 @@ class SettingsFragment : PreferenceFragmentCompat(), SharedPreferences.OnSharedP
|
||||
}
|
||||
|
||||
override fun showError(text: String, error: Throwable) {
|
||||
(activity as? BaseActivity)?.showError(text, error)
|
||||
(activity as? BaseActivity<*>)?.showError(text, error)
|
||||
}
|
||||
|
||||
override fun showMessage(text: String) {
|
||||
(activity as? BaseActivity)?.showMessage(text)
|
||||
(activity as? BaseActivity<*>)?.showMessage(text)
|
||||
}
|
||||
|
||||
override fun showExpiredDialog() {
|
||||
(activity as? BaseActivity<*>)?.showExpiredDialog()
|
||||
}
|
||||
|
||||
override fun openClearLoginView() {
|
||||
(activity as? BaseActivity<*>)?.openClearLoginView()
|
||||
}
|
||||
|
||||
override fun onResume() {
|
||||
|
@ -2,22 +2,26 @@ package io.github.wulkanowy.ui.modules.settings
|
||||
|
||||
import com.readystatesoftware.chuck.api.ChuckCollector
|
||||
import io.github.wulkanowy.data.repositories.preferences.PreferencesRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.services.sync.SyncManager
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.isHolidays
|
||||
import org.threeten.bp.LocalDate.now
|
||||
import timber.log.Timber
|
||||
import javax.inject.Inject
|
||||
|
||||
class SettingsPresenter @Inject constructor(
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val preferencesRepository: PreferencesRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper,
|
||||
private val syncManager: SyncManager,
|
||||
private val chuckCollector: ChuckCollector
|
||||
) : BasePresenter<SettingsView>(errorHandler) {
|
||||
) : BasePresenter<SettingsView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: SettingsView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -8,10 +8,10 @@ import io.github.wulkanowy.ui.modules.login.LoginActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import javax.inject.Inject
|
||||
|
||||
class SplashActivity : BaseActivity(), SplashView {
|
||||
class SplashActivity : BaseActivity<SplashPresenter>(), SplashView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: SplashPresenter
|
||||
override lateinit var presenter: SplashPresenter
|
||||
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
@ -31,9 +31,4 @@ class SplashActivity : BaseActivity(), SplashView {
|
||||
override fun showError(text: String, error: Throwable) {
|
||||
Toast.makeText(this, text, LENGTH_LONG).show()
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
presenter.onDetachView()
|
||||
super.onDestroy()
|
||||
}
|
||||
}
|
||||
|
@ -7,10 +7,10 @@ import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import javax.inject.Inject
|
||||
|
||||
class SplashPresenter @Inject constructor(
|
||||
private val studentRepository: StudentRepository,
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val schedulers: SchedulersProvider
|
||||
) : BasePresenter<SplashView>(errorHandler) {
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository
|
||||
) : BasePresenter<SplashView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
override fun onAttachView(view: SplashView) {
|
||||
super.onAttachView(view)
|
||||
|
@ -13,7 +13,7 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.Timetable
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.ui.modules.timetable.completed.CompletedLessonsFragment
|
||||
@ -21,7 +21,7 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_timetable.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class TimetableFragment : BaseSessionFragment(), TimetableView, MainView.MainChildView, MainView.TitledView {
|
||||
class TimetableFragment : BaseFragment(), TimetableView, MainView.MainChildView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: TimetablePresenter
|
||||
|
@ -5,8 +5,8 @@ import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.data.repositories.timetable.TimetableRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.isHolidays
|
||||
@ -22,13 +22,13 @@ import java.util.concurrent.TimeUnit.MILLISECONDS
|
||||
import javax.inject.Inject
|
||||
|
||||
class TimetablePresenter @Inject constructor(
|
||||
private val errorHandler: SessionErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val timetableRepository: TimetableRepository,
|
||||
private val studentRepository: StudentRepository,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<TimetableView>(errorHandler) {
|
||||
) : BasePresenter<TimetableView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var currentDate: LocalDate
|
||||
private set
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.timetable
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.Timetable
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface TimetableView : BaseSessionView {
|
||||
interface TimetableView : BaseView {
|
||||
|
||||
val roomString: String
|
||||
|
||||
|
@ -3,13 +3,13 @@ package io.github.wulkanowy.ui.modules.timetable.completed
|
||||
import android.content.res.Resources
|
||||
import com.readystatesoftware.chuck.api.ChuckCollector
|
||||
import io.github.wulkanowy.api.interceptor.FeatureDisabledException
|
||||
import io.github.wulkanowy.ui.base.session.SessionErrorHandler
|
||||
import io.github.wulkanowy.ui.base.ErrorHandler
|
||||
import javax.inject.Inject
|
||||
|
||||
class CompletedLessonsErrorHandler @Inject constructor(
|
||||
resources: Resources,
|
||||
chuckCollector: ChuckCollector
|
||||
) : SessionErrorHandler(resources, chuckCollector) {
|
||||
) : ErrorHandler(resources, chuckCollector) {
|
||||
|
||||
var onFeatureDisabled: () -> Unit = {}
|
||||
|
||||
|
@ -10,14 +10,14 @@ import eu.davidea.flexibleadapter.common.SmoothScrollLinearLayoutManager
|
||||
import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.R
|
||||
import io.github.wulkanowy.data.db.entities.CompletedLesson
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionFragment
|
||||
import io.github.wulkanowy.ui.base.BaseFragment
|
||||
import io.github.wulkanowy.ui.modules.main.MainActivity
|
||||
import io.github.wulkanowy.ui.modules.main.MainView
|
||||
import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.fragment_timetable_completed.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class CompletedLessonsFragment : BaseSessionFragment(), CompletedLessonsView, MainView.TitledView {
|
||||
class CompletedLessonsFragment : BaseFragment(), CompletedLessonsView, MainView.TitledView {
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: CompletedLessonsPresenter
|
||||
|
@ -5,7 +5,7 @@ import eu.davidea.flexibleadapter.items.AbstractFlexibleItem
|
||||
import io.github.wulkanowy.data.repositories.completedlessons.CompletedLessonsRepository
|
||||
import io.github.wulkanowy.data.repositories.semester.SemesterRepository
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionPresenter
|
||||
import io.github.wulkanowy.ui.base.BasePresenter
|
||||
import io.github.wulkanowy.utils.FirebaseAnalyticsHelper
|
||||
import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import io.github.wulkanowy.utils.isHolidays
|
||||
@ -21,13 +21,13 @@ import java.util.concurrent.TimeUnit
|
||||
import javax.inject.Inject
|
||||
|
||||
class CompletedLessonsPresenter @Inject constructor(
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val errorHandler: CompletedLessonsErrorHandler,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
studentRepository: StudentRepository,
|
||||
private val completedLessonsErrorHandler: CompletedLessonsErrorHandler,
|
||||
private val semesterRepository: SemesterRepository,
|
||||
private val completedLessonsRepository: CompletedLessonsRepository,
|
||||
private val analytics: FirebaseAnalyticsHelper
|
||||
) : BaseSessionPresenter<CompletedLessonsView>(errorHandler) {
|
||||
) : BasePresenter<CompletedLessonsView>(completedLessonsErrorHandler, studentRepository, schedulers) {
|
||||
|
||||
lateinit var currentDate: LocalDate
|
||||
private set
|
||||
@ -38,7 +38,7 @@ class CompletedLessonsPresenter @Inject constructor(
|
||||
view.initView()
|
||||
loadData(ofEpochDay(date ?: now().nextOrSameSchoolDay.toEpochDay()))
|
||||
reloadView()
|
||||
errorHandler.onFeatureDisabled = {
|
||||
completedLessonsErrorHandler.onFeatureDisabled = {
|
||||
this.view?.showFeatureDisabled()
|
||||
Timber.i("Completed lessons feature disabled by school")
|
||||
}
|
||||
@ -97,7 +97,7 @@ class CompletedLessonsPresenter @Inject constructor(
|
||||
}) {
|
||||
Timber.i("Loading completed lessons result: An exception occurred")
|
||||
view?.run { showEmpty(isViewEmpty) }
|
||||
errorHandler.dispatch(it)
|
||||
completedLessonsErrorHandler.dispatch(it)
|
||||
})
|
||||
}
|
||||
}
|
||||
|
@ -1,9 +1,9 @@
|
||||
package io.github.wulkanowy.ui.modules.timetable.completed
|
||||
|
||||
import io.github.wulkanowy.data.db.entities.CompletedLesson
|
||||
import io.github.wulkanowy.ui.base.session.BaseSessionView
|
||||
import io.github.wulkanowy.ui.base.BaseView
|
||||
|
||||
interface CompletedLessonsView : BaseSessionView {
|
||||
interface CompletedLessonsView : BaseView {
|
||||
|
||||
val isViewEmpty: Boolean
|
||||
|
||||
|
@ -18,13 +18,14 @@ import io.github.wulkanowy.utils.setOnItemClickListener
|
||||
import kotlinx.android.synthetic.main.activity_widget_configure.*
|
||||
import javax.inject.Inject
|
||||
|
||||
class TimetableWidgetConfigureActivity : BaseActivity(), TimetableWidgetConfigureView {
|
||||
class TimetableWidgetConfigureActivity : BaseActivity<TimetableWidgetConfigurePresenter>(),
|
||||
TimetableWidgetConfigureView {
|
||||
|
||||
@Inject
|
||||
lateinit var configureAdapter: FlexibleAdapter<AbstractFlexibleItem<*>>
|
||||
|
||||
@Inject
|
||||
lateinit var presenter: TimetableWidgetConfigurePresenter
|
||||
override lateinit var presenter: TimetableWidgetConfigurePresenter
|
||||
|
||||
override fun onCreate(savedInstanceState: Bundle?) {
|
||||
super.onCreate(savedInstanceState)
|
||||
@ -71,9 +72,4 @@ class TimetableWidgetConfigureActivity : BaseActivity(), TimetableWidgetConfigur
|
||||
override fun openLoginView() {
|
||||
startActivity(LoginActivity.getStartIntent(this))
|
||||
}
|
||||
|
||||
override fun onDestroy() {
|
||||
super.onDestroy()
|
||||
presenter.onDetachView()
|
||||
}
|
||||
}
|
||||
|
@ -11,11 +11,11 @@ import io.github.wulkanowy.utils.SchedulersProvider
|
||||
import javax.inject.Inject
|
||||
|
||||
class TimetableWidgetConfigurePresenter @Inject constructor(
|
||||
private val errorHandler: ErrorHandler,
|
||||
private val schedulers: SchedulersProvider,
|
||||
private val studentRepository: StudentRepository,
|
||||
schedulers: SchedulersProvider,
|
||||
errorHandler: ErrorHandler,
|
||||
studentRepository: StudentRepository,
|
||||
private val sharedPref: SharedPrefHelper
|
||||
) : BasePresenter<TimetableWidgetConfigureView>(errorHandler) {
|
||||
) : BasePresenter<TimetableWidgetConfigureView>(errorHandler, studentRepository, schedulers) {
|
||||
|
||||
private var appWidgetId: Int? = null
|
||||
|
||||
|
@ -1,5 +1,7 @@
|
||||
package io.github.wulkanowy.ui.modules.login
|
||||
|
||||
import io.github.wulkanowy.TestSchedulersProvider
|
||||
import io.github.wulkanowy.data.repositories.student.StudentRepository
|
||||
import org.junit.Assert.assertNotEquals
|
||||
import org.junit.Before
|
||||
import org.junit.Test
|
||||
@ -17,6 +19,9 @@ class LoginPresenterTest {
|
||||
@Mock
|
||||
lateinit var errorHandler: LoginErrorHandler
|
||||
|
||||
@Mock
|
||||
lateinit var studentRepository: StudentRepository
|
||||
|
||||
private lateinit var presenter: LoginPresenter
|
||||
|
||||
@Before
|
||||
@ -24,7 +29,7 @@ class LoginPresenterTest {
|
||||
MockitoAnnotations.initMocks(this)
|
||||
clearInvocations(loginView)
|
||||
|
||||
presenter = LoginPresenter(errorHandler)
|
||||
presenter = LoginPresenter(TestSchedulersProvider(), errorHandler, studentRepository)
|
||||
presenter.onAttachView(loginView)
|
||||
}
|
||||
|
||||
|
@ -39,7 +39,7 @@ class LoginFormPresenterTest {
|
||||
fun initPresenter() {
|
||||
MockitoAnnotations.initMocks(this)
|
||||
clearInvocations(repository, loginFormView)
|
||||
presenter = LoginFormPresenter(TestSchedulersProvider(), errorHandler, repository, analytics, false)
|
||||
presenter = LoginFormPresenter(TestSchedulersProvider(), repository, errorHandler, analytics, false)
|
||||
presenter.onAttachView(loginFormView)
|
||||
}
|
||||
|
||||
|
@ -40,7 +40,7 @@ class LoginStudentSelectPresenterTest {
|
||||
fun initPresenter() {
|
||||
MockitoAnnotations.initMocks(this)
|
||||
clearInvocations(studentRepository, loginStudentSelectView)
|
||||
presenter = LoginStudentSelectPresenter(errorHandler, studentRepository, TestSchedulersProvider(), analytics)
|
||||
presenter = LoginStudentSelectPresenter(TestSchedulersProvider(), studentRepository, errorHandler, analytics)
|
||||
presenter.onAttachView(loginStudentSelectView, null)
|
||||
}
|
||||
|
||||
|
@ -40,7 +40,7 @@ class MainPresenterTest {
|
||||
MockitoAnnotations.initMocks(this)
|
||||
clearInvocations(mainView)
|
||||
|
||||
presenter = MainPresenter(errorHandler, studentRepository, prefRepository, syncManager, TestSchedulersProvider(), analytics)
|
||||
presenter = MainPresenter(TestSchedulersProvider(), errorHandler, studentRepository, prefRepository, syncManager, analytics)
|
||||
presenter.onAttachView(mainView, null)
|
||||
}
|
||||
|
||||
|
@ -27,7 +27,7 @@ class SplashPresenterTest {
|
||||
@Before
|
||||
fun initPresenter() {
|
||||
MockitoAnnotations.initMocks(this)
|
||||
presenter = SplashPresenter(studentRepository, errorHandler, TestSchedulersProvider())
|
||||
presenter = SplashPresenter(TestSchedulersProvider(), errorHandler, studentRepository)
|
||||
}
|
||||
|
||||
@Test
|
||||
|
Reference in New Issue
Block a user